rotate
2024年06月19日
一、认识
CanvasRenderingContext2D.rotate()
是 Canvas 2D API
在变换矩阵中增加旋转的方法。角度变量表示一个顺时针旋转角度并且用弧度表示。
注意: 旋转中心点一直是 canvas
的起始点。如果想改变中心点,我们可以通过 translate()
方法移动 canvas
。如下图所示,以 canvas
起始点为中心,顺时针旋转 α
角度
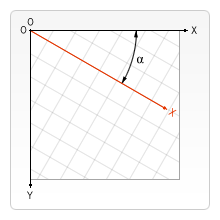
Preview
二、语法
const canvas = document.createElement('canvas');
const ctx = canvas.getContext('2d');
ctx.rotate(angle);
angle
: 顺时针旋转的弧度。如果你想通过角度值计算,可以使用公式:degree * Math.PI / 180
。
注意: rotate()
与 transform()
的关系如下:
const canvas = document.createElement('canvas');
const ctx = canvas.getContext('2d');
const degress = angle * (Math.PI / 180);
const cos = Math.cos(degress);
const sin = Math.sin(degress);
ctx.transform(cos, sin, -sin, cos, 0, 0);
等效于
const canvas = document.createElement('canvas');
const ctx = canvas.getContext('2d');
const degress = angle * (Math.PI / 180);
ctx.rotate(degress);
三、场景
3.1 绘制矩形, 并以起始点为中心, 旋转 30 度
function drawRotateRectangle(params) {
const canvas = document.createElement("canvas");
const ctx = canvas.getContext("2d");
canvas.width = 400;
canvas.height = 400;
// 旋转前 绘制矩形
ctx.fillStyle = "grey";
ctx.fillRect(200, 0, 100, 50);
// 旋转画布
ctx.rotate(30 * (Math.PI / 180));
// 旋转后 绘制矩形
ctx.fillStyle = "red";
ctx.fillRect(200, 0, 100, 50);
// 旋转画布
ctx.rotate(32 * (Math.PI / 180));
// 旋转后 绘制矩形
ctx.fillStyle = "blue";
ctx.fillRect(200, 0, 100, 50);
document.body.appendChild(canvas);
}
drawRotateRectangle();
3.2 绘制文本, 并以起始点为中心, 旋转 -30 度
function drawRotateText(params) {
const { text, angle = -30 } = params || {};
const canvas = document.createElement("canvas");
const ctx = canvas.getContext("2d");
canvas.width = 180;
canvas.height = 100;
// 先旋转
ctx.rotate(angle * (Math.PI / 180));
// 再绘制
ctx.fillStyle = "#000";
ctx.font = `16px serif`;
ctx.fillText(text, 0, 80);
document.body.appendChild(canvas);
}
drawRotateText({
text: "旋转文案",
});
3.3 更改旋转中心, 并保存起始点, 并旋转 30 度
const canvas = document.createElement("canvas");
const ctx = canvas.getContext("2d");
// 保存当前 Canvas 状态, 当前状态是 (0, 0) 原点
ctx.save();
// 更改 Canvas 旋转中心为 Canvas 中心, 此时已经将 Canvas 起始点也更改为 Canvas 中心点
ctx.translate(canvas.width / 2, canvas.height / 2);
ctx.rotate(30 * (Math.PI / 180));
ctx.fillStyle = "red";
ctx.fillRect(0, 0, 50, 50);
// 恢复到上一个 Canvas 状态, 即 (0, 0) 原点
ctx.restore();
ctx.fillStyle = "blue";
ctx.fillRect(0, 0, 50, 50);
document.body.appendChild(canvas);